06. Getting User Data in Flask — Part 1
Getting user data in Flask Heading
Getting User Data in Flask — Part 1
Methods of getting user data from the view (in Flask)
ND004 C01 L05 06 Getting User Data In Flask
Takeaways
-
There are 3 methods of getting user data from a view to a controller. See the image below.
- URL query parameters
- Forms
- JSON
URL query parameters
-
URL query parameters are listed as key-value pairs at the end of a URL, preceding a "?" question mark. E.g.
www.example.com/hello?my_key=my_value
.
Form data
-
request.form.get('<name>')
reads thevalue
from a form input control (text input, number input, password input, etc) by thename
attribute on the input HTML element.
Note: defaults
-
request.args.get
,request.form.get
both accept an optional second parameter, e.g.request.args.get('foo', 'my default')
, set to a default value, in case the result is empty.
JSON
-
request.data
retrieves JSON as a string . Then we'd take that string and turn it into python constructs by callingjson.loads
on therequest.data
string to turn it into lists and dictionaries in Python.
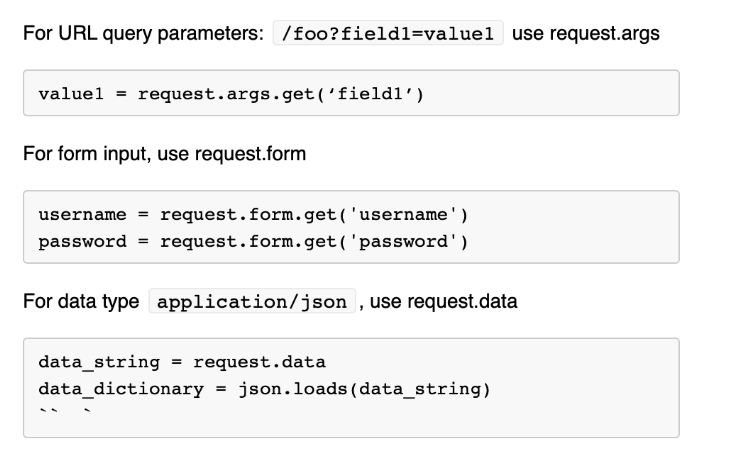
Three methods of getting user data in Flask
Input data quiz
QUIZ QUESTION: :
Match the flask method to the type of user data being handled
ANSWER CHOICES:
Flask Method |
User Input |
---|---|
HTTP |
|
JSON |
|
Form input |
|
URL query parameters |
SOLUTION:
Flask Method |
User Input |
---|---|
JSON |
|
Form input |
|
URL query parameters |
Getting user data in Flask Recap
Using HTML form submission to get the data
ND004 C01 L05 06.1 Getting User Data In Flask
Takeaways
-
forms take an
action
(name of the route) andmethod
(route method) to submit data to our server. -
The
name
attribute on a form control element is the key used to retrieve data fromrequest.get(<key>)
. - All forms either define a submit button, or allow the user to hit ENTER on an input to submit the form.
SOLUTION:
bar2
Form methods
POST
vs
GET
ND004 C01 L05 06.2 Getting User Data In Flask
Video Correction Notes
- "Response Body" should be corrected to "Request Body", throughout, since the client is sending off a request .
Takeaways
- The way form data traverses from the client to server differs based on whether we are using a GET or a POST method on the form.
The POST submission
-
On submit, we send off an HTTP POST request to the route
/create
with a request body -
The request body stringifies the key-value pairs of fields from the form (as part of the
name
attribute) along with their values.
The GET submission
- Sends off a GET request with URL query parameters that appends the form data to the URL.
- Ideal for smaller form submissions.
POSTs are ideal for longer form submissions, since URL query parameters can only be so long compared to request bodies (max 2048 characters).
Moreover, forms can only send POST and GET requests, and nothing else.
Methods Quiz
QUIZ QUESTION: :
Match the HTTP request route to the type of form submission it was
ANSWER CHOICES:
Request |
Form Submission |
---|---|
A POST form submission |
|
A GET form submission |
|
A PUT form submission |
SOLUTION:
Request |
Form Submission |
---|---|
A POST form submission |
|
A GET form submission |